Common Use Cases for JSON
What Are Top 3 Common Uses Cases For JSON with examples?
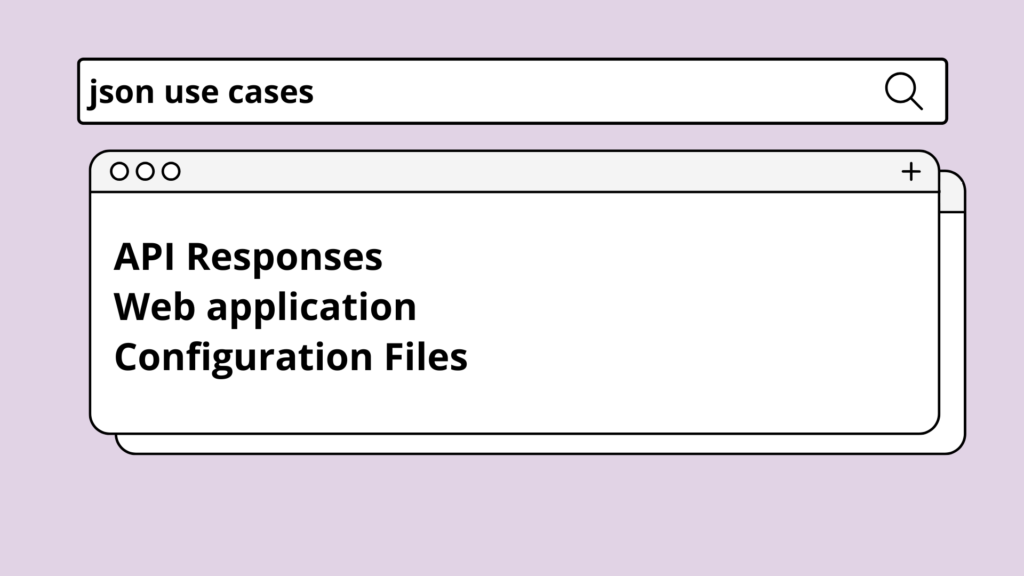
In this blog we will look into
1 API responses with JSON
One of the most common use cases for JSON is in API responses. When a client makes a request to a server, the server often responds with data formatted in JSON, allowing for seamless integration.
When it comes to working with APIs, JSON is the format that simplifies data exchange between servers and clients! Let’s dive into an exciting use case that showcases how JSON can streamline API responses, making your coding experience in Ruby and Python a breeze!
Imagine you’re developing a weather application that fetches real-time data from a weather API. When you send a request to the API, it responds with a treasure trove of information in JSON format. Here’s an example of what that response might look like:
{
“location”: “New York”,
“temperature”: {
“current”: 75,
“high”: 80,
“low”: 65
},
“conditions”: “Sunny”
}
In Ruby
In Ruby, you can easily parse this response using the `json` library:
require ‘json’
require ‘net/http’
uri = URI(‘https://api.weather.com/v3/weather/current?city=NewYork’)
response = Net::HTTP.get(uri)
weather_data = JSON.parse(response)
puts “The current temperature in #{weather_data[‘location’]} is #{weather_data[‘temperature’][‘current’]}°F and it’s #{weather_data[‘conditions’]}!”
In Python
And for Python enthusiasts, here’s how you can achieve the same thing using the popular `requests` library:
import requests
response = requests.get(‘https://api.weather.com/v3/weather/current?city=NewYork’)
weather_data = response.json()
print(f”The current temperature in {weather_data[‘location’]} is {weather_data[‘temperature’][‘current’]}°F and it’s {weather_data[‘conditions’]}!”)
With just a few lines of code, both Ruby and Python make it super simple to retrieve and display dynamic data from an API. This use case not only highlights the versatility of JSON but also demonstrates how easily developers can integrate external data into their applications.
2 Web applications using JSON
Imagine you’re building a dynamic web application using Ruby on Rails for the backend and Python for some nifty data processing tasks. You want to fetch user information from your database and display it on the front end without reloading the entire page. This is where JSON shines!
In Ruby on Rails
In your Ruby on Rails controller, you can easily convert user data into JSON format. Here’s a quick example:
def index
@users = User.all
render json: @users
end
In Python's Flask
This simple line of code transforms all user records into a JSON array! Now, on the client side, you can use Python with frameworks like Flask to make an AJAX call to this endpoint:
fetch(‘/users’)
.then(response => response.json())
.then(data => {
console.log(data); // Here’s your user data in JSON format!
// Code to dynamically render users goes here!
});
3.Configuration files
Imagine you’re working on a web application using Ruby’s Ruby on Rails or Python’s Flask. You need to manage settings like database connections, API keys, or feature toggles. Instead of hardcoding these values into your codebase—making changes cumbersome and risky—you can create a JSON configuration file.
{
“database”: {
“host”: “localhost”,
“port”: 5432,
“username”: “user”,
“password”: “pass”
},
“apiKeys”: {
“serviceA”: “abc123”,
“serviceB”: “xyz789”
},
“features”: {
“enableFeatureX”: true,
“enableFeatureY”: false
}
}
In Ruby on Rails
In Ruby, you can easily parse this JSON file using the `json` gem:
require ‘json’
file = File.read(‘config.json’)
config = JSON.parse(file)
puts config[‘database’][‘host’] # Outputs: localhost
In Python
In Python, the process is just as straightforward with the built-in `json` module:
import json
with open(‘config.json’) as f:
config = json.load(f)
print(config[‘apiKeys’][‘serviceA’]) # Outputs: abc123
In Javascript
And for JavaScript just fetch and parse with ease
fetch(‘config.json’)
.then(response => response.json())
.then(config => console.log(config.features.enableFeatureX)); // Outputs: true